cb.onDrawPanel(func)¶
This function is only available to apps, not bots.
Return data needed to display the info panel for a user. The func
argument should be a function that receives 1 argument itself, user.
The return value is a key-value set with a template
key. Depending on the
template chosen, additional keys should be passed in. For more information,
see Available Templates
Available Templates¶
These are the supported templates with examples of how to use them.
3_rows_of_labels¶
3_rows_of_labels consists of three rows of data, each row of the template includes labels and values.
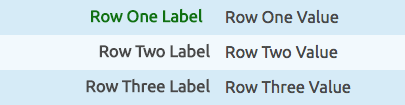
Example
/* Layout is roughly
{row1_label}: {row1_value}
{row2_label}: {row2_value}
{row3_label}: {row3_value}
*/
cb.onDrawPanel(function(user) {
return {
'template': '3_rows_of_labels',
'row1_label': 'Tip Received / Goal :',
'row1_value': '0',
'row2_label': 'Highest Tip:',
'row2_value': user['user'],
'row3_label': 'Latest Tip Received:',
'row3_value': '0'
};
});
3_rows_11_21_31¶
3_rows_11_21_31 consists of three rows of data, each row of the template includes only values.
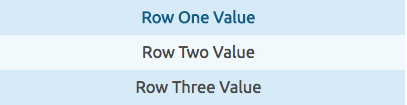
Example
/* Layout is roughly
{row1_value}
{row2_value}
{row3_value}
*/
cb.onDrawPanel(function(user) {
return {
'template': '3_rows_11_21_31',
'row1_value': '0',
'row2_value': user['user'],
'row3_value': '0'
};
});
3_rows_12_21_31¶
3_rows_12_21_31 consists of three rows of data. The first row will includes both label and value. The second and third rows includes only values.
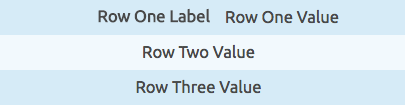
Example
/* Layout is roughly
{row1_label}: {row1_value}
{row2_value}
{row3_value}
*/
cb.onDrawPanel(function(user) {
return {
'template': '3_rows_12_21_31',
'row1_label': 'Tip Received / Goal :',
'row1_value': '0',
'row2_value': user['user'],
'row3_value': '0'
};
});
3_rows_12_22_31¶
3_rows_12_22_31 consists of three rows of data. The first and second rows include both labelS and valueS. The third row includes only value.
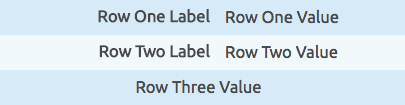
Example
/* Layout is roughly
{row1_label}: {row1_value}
{row2_label}: {row2_value}
{row3_value}
*/
cb.onDrawPanel(function(user) {
return {
'template': '3_rows_12_22_31',
'row1_label': 'Tip Received / Goal :',
'row1_value': '0',
'row2_label': 'Highest Tip:',
'row2_value': user['user'],
'row3_value': '0'
};
});
image_template¶
image_template offers the ability to pass a list of objects to be added to the app panel.
The two types of objects are image
add text
.
Each object is applied to the panel in the order specified by the layers
list.
The dimensions of the app panel are 270 X 69 pixels; text and images that overflow the app panel will be hidden.
image_template also offers the ability to include a customizable table. The table has three rows and each row can consist of one or two columns. The height of each row is 23px and cannot be changed. Rows that are omitted will be left blank. A limited amount of css styling can be specified in the template. The font-family and font-size of the table are not configurable, the defaults are UbuntuRegular and 11px.
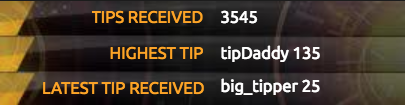
Example and Documentation
- Image
- Required fields:
type: ‘image’
fileID: ID that was assigned to file during upload
- Optional fields:
left [default=0, min=0, max=270]: number of pixels from the left of the app panel that the top, left corner of the image will be placed.
top [default=0, min=0, max=69]: number of pixels from the top of the app panel that the top, left corner of the image will be placed.
opacity [default=1]: specifies the opacity of image from 0.0 (fully transparent) to 1.0 (fully opaque)
- Text
- Required fields:
type: ‘text’
- Optional fields:
color [default=black]: color of the text.
font-family [default=UbuntuRegular]: font-family of the text.
font-size [default=11]: font-size of the text in pixels.
font-style [default=normal]: font-style of the text.
left [default=0, min=0, max=270]: number of pixels from the left of the app panel that the top, left corner of the text element will be placed.
top [default=0, min=0, max=69]:: number of pixels from the top of the app panel that the top, left corner of the text element will be placed.
text [default=””]: text to be displayed.
width [max=270]: width of the text element, overflow will be hidden and ellipsis will be shown.
max-width [max=270]: max-width of the text element, overflow will be hidden and ellipsis will be shown.
- Table
- Required fields:
type: ‘table’
- Optional fields (order of inheritance is table, row, header/data):
color [default=black]: color of the text for the table.
background-color [default=None]: color of the background for the table
text-align [default=center]: alignment of the text for the table
font-weight [default=normal]: weight of the font for the table
font-style [default=normal]: style of the font for the table
width (header only) [default=135]: width of the header column in pixels
- Uploading an Image File
Go to the
edit
tab on the app pageClick Upload Image Files (or Manage Image Files if you have already uploaded an image)
Select one or more files and click upload
If upload successful you will be redirect to the file management page
Each file will have a unique ID
The ID should be used as the fileID in the image layer
Image for example code background_image.jpg
var backgroundImage = '05b83220-1ccc-4871-9333-70f97488de00';
var tipsReceived = 3545;
var highestTip = 'tipDaddy 135';
var lastTipReceived = 'big_tipper 25';
var fontSize = 11;
cb.onDrawPanel(function(user) {
return {
"template": "image_template",
"layers": [
{'type': 'image', 'fileID': backgroundImage},
{
'type': 'text',
'text': 'TIPS RECEIVED',
'top': 5,
'left': 61,
'font-size': fontSize,
'color': 'orange',
},
{
'type': 'text',
'text': 'HIGHEST TIP',
'top': 29,
'left': 73,
'font-size': fontSize,
'color': 'orange',
},
{
'type': 'text',
'text': 'LATEST TIP RECEIVED',
'top': 52,
'left': 28,
'font-size': fontSize,
'color': 'orange',
},
{
'type': 'text',
'text': tipsReceived,
'top': 5,
'left': 147,
'font-size': fontSize,
'color': 'white',
},
{
'type': 'text',
'text': highestTip,
'top': 29,
'left': 147,
'font-size': fontSize,
'color': 'white',
},
{
'type': 'text',
'text': lastTipReceived,
'top': 51,
'left': 147,
'font-size': fontSize,
'color': 'white',
},
],
};
});
cb.drawPanel();
Additional templates¶
If nothing here works for you, please email support and request an additional template to suit your needs. Let us know what you’re looking for and we’ll try to accommodate you.